Load ODIM_H5 Volume data from German Weather Service#
In this example, we obtain and read the latest 30 minutes of available volumetric radar data from German Weather Service available at opendata.dwd.de. Finally we do some plotting.
This retrieves 6 timesteps of the 10 sweeps (moments DBZH and VRADH) of the DWD volume scan of a distinct radar. This amounts to 120 data files which are combined into one volumetric Cf/Radial2 like xarray powered structure.
Exports to single file Odim_H5 and Cf/Radial2 format are shown at the end of this tutorial.
Note
The following code is based on xarray, xarray-datatree and xradar. It claims multiple data files and presents them in a DataTree
.
[1]:
import wradlib as wrl
import warnings
warnings.filterwarnings("ignore")
import matplotlib.pyplot as plt
import numpy as np
import xarray as xr
try:
get_ipython().run_line_magic("matplotlib inline")
except:
plt.ion()
/home/runner/micromamba/envs/wradlib-tests/lib/python3.11/site-packages/h5py/__init__.py:36: UserWarning: h5py is running against HDF5 1.14.3 when it was built against 1.14.2, this may cause problems
_warn(("h5py is running against HDF5 {0} when it was built against {1}, "
[2]:
import urllib3
import os
import io
import glob
import shutil
import datetime
Download radar volumes of latest 30 minutes from server using wetterdienst
#
wetterdienst
is a neat package for easy retrieval of data primarily from DWD. For further information have a look at their documentation.
[3]:
from wetterdienst.provider.dwd.radar import (
DwdRadarDataFormat,
DwdRadarDataSubset,
DwdRadarParameter,
DwdRadarValues,
)
from wetterdienst.provider.dwd.radar.sites import DwdRadarSite
[4]:
elevations = range(10)
end_date = datetime.datetime.utcnow()
start_date = end_date - datetime.timedelta(minutes=30)
results_velocity = []
results_reflectivity = []
for el in elevations:
# Horizontal Doppler Velocity
request_velocity = DwdRadarValues(
parameter=DwdRadarParameter.SWEEP_VOL_VELOCITY_H,
start_date=start_date,
end_date=end_date,
site=DwdRadarSite.ESS,
elevation=el,
fmt=DwdRadarDataFormat.HDF5,
subset=DwdRadarDataSubset.POLARIMETRIC,
)
# Horizontal Reflectivity
request_reflectivity = DwdRadarValues(
parameter=DwdRadarParameter.SWEEP_VOL_REFLECTIVITY_H,
start_date=start_date,
end_date=end_date,
elevation=el,
site=DwdRadarSite.ESS,
fmt=DwdRadarDataFormat.HDF5,
subset=DwdRadarDataSubset.POLARIMETRIC,
)
# Submit requests.
results_velocity.append(request_velocity.query())
results_reflectivity.append(request_reflectivity.query())
[5]:
import wetterdienst
wetterdienst.__version__
[5]:
'0.49.0'
Acquire data as memory buffer#
[6]:
%%time
volume_velocity = []
for item1 in results_velocity:
files = []
for item2 in item1:
files.append(item2.data)
volume_velocity.append(files)
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:02<08:46, 2.20s/it]
</pre>
- 0%| | 1/240 [00:02<08:46, 2.20s/it]
end{sphinxVerbatim}
0%| | 1/240 [00:02<08:46, 2.20s/it]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:02<03:57, 1.00it/s]
</pre>
- 1%| | 2/240 [00:02<03:57, 1.00it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:02<03:57, 1.00it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:02<02:24, 1.64it/s]
</pre>
- 1%|▏ | 3/240 [00:02<02:24, 1.64it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:02<02:24, 1.64it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:02<01:40, 2.34it/s]
</pre>
- 2%|▏ | 4/240 [00:02<01:40, 2.34it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:02<01:40, 2.34it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:02<01:16, 3.08it/s]
</pre>
- 2%|▏ | 5/240 [00:02<01:16, 3.08it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:02<01:16, 3.08it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:02<01:01, 3.80it/s]
</pre>
- 2%|▎ | 6/240 [00:02<01:01, 3.80it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:02<01:01, 3.80it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:03<00:52, 4.47it/s]
</pre>
- 3%|▎ | 7/240 [00:03<00:52, 4.47it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:03<00:52, 4.47it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:03<01:42, 2.27it/s]
</pre>
- 3%|▎ | 7/240 [00:03<01:42, 2.27it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:03<01:42, 2.27it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:21, 1.69it/s]
</pre>
- 0%| | 1/240 [00:00<02:21, 1.69it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:21, 1.69it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:18, 3.03it/s]
</pre>
- 1%| | 2/240 [00:00<01:18, 3.03it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:18, 3.03it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:58, 4.07it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:58, 4.07it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:58, 4.07it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:01<00:48, 4.86it/s]
</pre>
- 2%|▏ | 4/240 [00:01<00:48, 4.86it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:01<00:48, 4.86it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:43, 5.46it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:43, 5.46it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:43, 5.46it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:39, 5.91it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:39, 5.91it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:39, 5.91it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:37, 6.25it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:37, 6.25it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:37, 6.25it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:48, 4.81it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:48, 4.81it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:48, 4.81it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:21, 1.69it/s]
</pre>
- 0%| | 1/240 [00:00<02:21, 1.69it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:21, 1.69it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:19, 3.01it/s]
</pre>
- 1%| | 2/240 [00:00<01:19, 3.01it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:19, 3.01it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<01:04, 3.65it/s]
</pre>
- 1%|▏ | 3/240 [00:00<01:04, 3.65it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<01:04, 3.65it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:01<00:52, 4.49it/s]
</pre>
- 2%|▏ | 4/240 [00:01<00:52, 4.49it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:01<00:52, 4.49it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:45, 5.21it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:45, 5.21it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:45, 5.21it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:40, 5.77it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:40, 5.77it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:40, 5.77it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:37, 6.26it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:37, 6.26it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:37, 6.26it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:49, 4.67it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:49, 4.67it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:49, 4.67it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:35, 1.54it/s]
</pre>
- 0%| | 1/240 [00:00<02:35, 1.54it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:35, 1.54it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:23, 2.84it/s]
</pre>
- 1%| | 2/240 [00:00<01:23, 2.84it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:23, 2.84it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<01:01, 3.88it/s]
</pre>
- 1%|▏ | 3/240 [00:00<01:01, 3.88it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<01:01, 3.88it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:01<00:51, 4.54it/s]
</pre>
- 2%|▏ | 4/240 [00:01<00:51, 4.54it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:01<00:51, 4.54it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:45, 5.19it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:45, 5.19it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:45, 5.19it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:41, 5.69it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:41, 5.69it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:41, 5.69it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:38, 6.08it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:38, 6.08it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:38, 6.08it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:50, 4.57it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:50, 4.57it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:50, 4.57it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:17, 1.74it/s]
</pre>
- 0%| | 1/240 [00:00<02:17, 1.74it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:17, 1.74it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:17, 3.06it/s]
</pre>
- 1%| | 2/240 [00:00<01:17, 3.06it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:17, 3.06it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:58, 4.08it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:58, 4.08it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:58, 4.08it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:01<00:48, 4.84it/s]
</pre>
- 2%|▏ | 4/240 [00:01<00:48, 4.84it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:01<00:48, 4.84it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:43, 5.39it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:43, 5.39it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:43, 5.39it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:40, 5.78it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:40, 5.78it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:40, 5.78it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:38, 6.09it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:38, 6.09it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:38, 6.09it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:48, 4.77it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:48, 4.77it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:48, 4.77it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:21, 1.69it/s]
</pre>
- 0%| | 1/240 [00:00<02:21, 1.69it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:21, 1.69it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:18, 3.03it/s]
</pre>
- 1%| | 2/240 [00:00<01:18, 3.03it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:18, 3.03it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:58, 4.04it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:58, 4.04it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:58, 4.04it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:01<00:49, 4.79it/s]
</pre>
- 2%|▏ | 4/240 [00:01<00:49, 4.79it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:01<00:49, 4.79it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:43, 5.35it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:43, 5.35it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:43, 5.35it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:40, 5.76it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:40, 5.76it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:40, 5.76it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:38, 6.07it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:38, 6.07it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:38, 6.07it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:49, 4.73it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:49, 4.73it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:49, 4.73it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:31, 1.58it/s]
</pre>
- 0%| | 1/240 [00:00<02:31, 1.58it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:31, 1.58it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:18, 3.02it/s]
</pre>
- 1%| | 2/240 [00:00<01:18, 3.02it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:18, 3.02it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:55, 4.30it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:55, 4.30it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:55, 4.30it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:44, 5.34it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:44, 5.34it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:44, 5.34it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:38, 6.18it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:38, 6.18it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:38, 6.18it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:34, 6.85it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:34, 6.85it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:34, 6.85it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:31, 7.38it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:31, 7.38it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:31, 7.38it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:44, 5.25it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:44, 5.25it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:44, 5.25it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:12, 1.80it/s]
</pre>
- 0%| | 1/240 [00:00<02:12, 1.80it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:12, 1.80it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:10, 3.38it/s]
</pre>
- 1%| | 2/240 [00:00<01:10, 3.38it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:10, 3.38it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:50, 4.72it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:50, 4.72it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:50, 4.72it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:40, 5.78it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:40, 5.78it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:40, 5.78it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:35, 6.63it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:35, 6.63it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:35, 6.63it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:32, 7.28it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:32, 7.28it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:32, 7.28it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:30, 7.76it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:30, 7.76it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:30, 7.76it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:40, 5.70it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:40, 5.70it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:40, 5.70it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:16, 1.76it/s]
</pre>
- 0%| | 1/240 [00:00<02:16, 1.76it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:16, 1.76it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:11, 3.33it/s]
</pre>
- 1%| | 2/240 [00:00<01:11, 3.33it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:11, 3.33it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:50, 4.67it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:50, 4.67it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:50, 4.67it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:40, 5.76it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:40, 5.76it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:40, 5.76it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:35, 6.60it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:35, 6.60it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:35, 6.60it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:32, 7.26it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:32, 7.26it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:32, 7.26it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:29, 7.77it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:29, 7.77it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:29, 7.77it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:41, 5.65it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:41, 5.65it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:41, 5.65it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:05, 1.90it/s]
</pre>
- 0%| | 1/240 [00:00<02:05, 1.90it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:05, 1.90it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:07, 3.54it/s]
</pre>
- 1%| | 2/240 [00:00<01:07, 3.54it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:07, 3.54it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:48, 4.89it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:48, 4.89it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:48, 4.89it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:39, 5.95it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:39, 5.95it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:39, 5.95it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:00<00:34, 6.79it/s]
</pre>
- 2%|▏ | 5/240 [00:00<00:34, 6.79it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:00<00:34, 6.79it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:31, 7.39it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:31, 7.39it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:31, 7.39it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:29, 7.85it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:29, 7.85it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:29, 7.85it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:39, 5.85it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:39, 5.85it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:39, 5.85it/s]
CPU times: user 3.48 s, sys: 122 ms, total: 3.6 s
Wall time: 15.5 s
[7]:
volume_velocity = [v[-6:] for v in volume_velocity]
volume_velocity = np.array(volume_velocity).T.tolist()
[8]:
%%time
volume_reflectivity = []
for item1 in results_reflectivity:
files = []
for item2 in item1:
files.append(item2.data)
volume_reflectivity.append(files)
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:12, 1.80it/s]
</pre>
- 0%| | 1/240 [00:00<02:12, 1.80it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:12, 1.80it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:11, 3.34it/s]
</pre>
- 1%| | 2/240 [00:00<01:11, 3.34it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:11, 3.34it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:51, 4.59it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:51, 4.59it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:51, 4.59it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:42, 5.58it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:42, 5.58it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:42, 5.58it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:37, 6.33it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:37, 6.33it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:37, 6.33it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:34, 6.87it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:34, 6.87it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:34, 6.87it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:32, 7.27it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:32, 7.27it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:32, 7.27it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:42, 5.47it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:42, 5.47it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:42, 5.47it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:10, 1.83it/s]
</pre>
- 0%| | 1/240 [00:00<02:10, 1.83it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:10, 1.83it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:10, 3.37it/s]
</pre>
- 1%| | 2/240 [00:00<01:10, 3.37it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:10, 3.37it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:51, 4.60it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:51, 4.60it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:51, 4.60it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:42, 5.53it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:42, 5.53it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:42, 5.53it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:37, 6.24it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:37, 6.24it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:37, 6.24it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:34, 6.80it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:34, 6.80it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:34, 6.80it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:32, 7.19it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:32, 7.19it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:32, 7.19it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:42, 5.45it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:42, 5.45it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:42, 5.45it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:14, 1.77it/s]
</pre>
- 0%| | 1/240 [00:00<02:14, 1.77it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:14, 1.77it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:13, 3.26it/s]
</pre>
- 1%| | 2/240 [00:00<01:13, 3.26it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:13, 3.26it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:53, 4.46it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:53, 4.46it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:53, 4.46it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:43, 5.39it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:43, 5.39it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:43, 5.39it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:38, 6.10it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:38, 6.10it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:38, 6.10it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:35, 6.61it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:35, 6.61it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:35, 6.61it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:33, 7.02it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:33, 7.02it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:33, 7.02it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:43, 5.30it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:43, 5.30it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:43, 5.30it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:16, 1.74it/s]
</pre>
- 0%| | 1/240 [00:00<02:16, 1.74it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:16, 1.74it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:15, 3.17it/s]
</pre>
- 1%| | 2/240 [00:00<01:15, 3.17it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:15, 3.17it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:55, 4.29it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:55, 4.29it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:55, 4.29it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:45, 5.14it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:45, 5.14it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:45, 5.14it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:40, 5.79it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:40, 5.79it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:40, 5.79it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:37, 6.25it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:37, 6.25it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:37, 6.25it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:35, 6.63it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:35, 6.63it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:35, 6.63it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:45, 5.07it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:45, 5.07it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:45, 5.07it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:15, 1.76it/s]
</pre>
- 0%| | 1/240 [00:00<02:15, 1.76it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:15, 1.76it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:16, 3.12it/s]
</pre>
- 1%| | 2/240 [00:00<01:16, 3.12it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:16, 3.12it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:01<02:11, 1.81it/s]
</pre>
- 1%|▏ | 3/240 [00:01<02:11, 1.81it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:01<02:11, 1.81it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:01<01:34, 2.51it/s]
</pre>
- 2%|▏ | 4/240 [00:01<01:34, 2.51it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:01<01:34, 2.51it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<01:13, 3.22it/s]
</pre>
- 2%|▏ | 5/240 [00:01<01:13, 3.22it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<01:13, 3.22it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:02<00:59, 3.91it/s]
</pre>
- 2%|▎ | 6/240 [00:02<00:59, 3.91it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:02<00:59, 3.91it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:02<00:51, 4.54it/s]
</pre>
- 3%|▎ | 7/240 [00:02<00:51, 4.54it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:02<00:51, 4.54it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:02<01:11, 3.24it/s]
</pre>
- 3%|▎ | 7/240 [00:02<01:11, 3.24it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:02<01:11, 3.24it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:20, 1.70it/s]
</pre>
- 0%| | 1/240 [00:00<02:20, 1.70it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:20, 1.70it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:19, 3.00it/s]
</pre>
- 1%| | 2/240 [00:00<01:19, 3.00it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:19, 3.00it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:59, 4.01it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:59, 4.01it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:59, 4.01it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:01<00:49, 4.77it/s]
</pre>
- 2%|▏ | 4/240 [00:01<00:49, 4.77it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:01<00:49, 4.77it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:44, 5.32it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:44, 5.32it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:44, 5.32it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:40, 5.74it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:40, 5.74it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:40, 5.74it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:38, 6.05it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:38, 6.05it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:38, 6.05it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:49, 4.71it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:49, 4.71it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:49, 4.71it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:11, 1.82it/s]
</pre>
- 0%| | 1/240 [00:00<02:11, 1.82it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:11, 1.82it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:10, 3.38it/s]
</pre>
- 1%| | 2/240 [00:00<01:10, 3.38it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:10, 3.38it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:50, 4.66it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:50, 4.66it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:50, 4.66it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:41, 5.67it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:41, 5.67it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:41, 5.67it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:36, 6.49it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:36, 6.49it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:36, 6.49it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:33, 7.07it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:33, 7.07it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:33, 7.07it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:31, 7.51it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:31, 7.51it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:31, 7.51it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:41, 5.59it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:41, 5.59it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:41, 5.59it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:10, 1.84it/s]
</pre>
- 0%| | 1/240 [00:00<02:10, 1.84it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:10, 1.84it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:09, 3.44it/s]
</pre>
- 1%| | 2/240 [00:00<01:09, 3.44it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:09, 3.44it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:49, 4.77it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:49, 4.77it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:49, 4.77it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:40, 5.84it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:40, 5.84it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:40, 5.84it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:00<00:35, 6.63it/s]
</pre>
- 2%|▏ | 5/240 [00:00<00:35, 6.63it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:00<00:35, 6.63it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:32, 7.20it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:32, 7.20it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:32, 7.20it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:30, 7.64it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:30, 7.64it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:30, 7.64it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:40, 5.70it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:40, 5.70it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:40, 5.70it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:11, 1.82it/s]
</pre>
- 0%| | 1/240 [00:00<02:11, 1.82it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:11, 1.82it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:09, 3.42it/s]
</pre>
- 1%| | 2/240 [00:00<01:09, 3.42it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:09, 3.42it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:49, 4.76it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:49, 4.76it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:49, 4.76it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:40, 5.81it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:40, 5.81it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:40, 5.81it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:01<00:35, 6.61it/s]
</pre>
- 2%|▏ | 5/240 [00:01<00:35, 6.61it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:01<00:35, 6.61it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:32, 7.27it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:32, 7.27it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:32, 7.27it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:30, 7.75it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:30, 7.75it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:30, 7.75it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:40, 5.71it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:40, 5.71it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:40, 5.71it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/240 [00:00<?, ?it/s]
</pre>
- 0%| | 0/240 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/240 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/240 [00:00<02:06, 1.89it/s]
</pre>
- 0%| | 1/240 [00:00<02:06, 1.89it/s]
end{sphinxVerbatim}
0%| | 1/240 [00:00<02:06, 1.89it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/240 [00:00<01:07, 3.51it/s]
</pre>
- 1%| | 2/240 [00:00<01:07, 3.51it/s]
end{sphinxVerbatim}
1%| | 2/240 [00:00<01:07, 3.51it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 3/240 [00:00<00:49, 4.83it/s]
</pre>
- 1%|▏ | 3/240 [00:00<00:49, 4.83it/s]
end{sphinxVerbatim}
1%|▏ | 3/240 [00:00<00:49, 4.83it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 4/240 [00:00<00:40, 5.88it/s]
</pre>
- 2%|▏ | 4/240 [00:00<00:40, 5.88it/s]
end{sphinxVerbatim}
2%|▏ | 4/240 [00:00<00:40, 5.88it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/240 [00:00<00:34, 6.73it/s]
</pre>
- 2%|▏ | 5/240 [00:00<00:34, 6.73it/s]
end{sphinxVerbatim}
2%|▏ | 5/240 [00:00<00:34, 6.73it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 6/240 [00:01<00:31, 7.37it/s]
</pre>
- 2%|▎ | 6/240 [00:01<00:31, 7.37it/s]
end{sphinxVerbatim}
2%|▎ | 6/240 [00:01<00:31, 7.37it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:29, 7.86it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:29, 7.86it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:29, 7.86it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 7/240 [00:01<00:40, 5.82it/s]
</pre>
- 3%|▎ | 7/240 [00:01<00:40, 5.82it/s]
end{sphinxVerbatim}
3%|▎ | 7/240 [00:01<00:40, 5.82it/s]
CPU times: user 3.32 s, sys: 69.7 ms, total: 3.39 s
Wall time: 13.8 s
[9]:
volume_reflectivity = [v[-6:] for v in volume_reflectivity]
volume_reflectivity = np.array(volume_reflectivity).T.tolist()
Read the data into xarray powered structure#
[10]:
from datatree import DataTree, open_datatree
import xradar
def concat_radar_datatree(objs, dim="volume_time"):
root_ds = [obj["/"].ds for obj in objs]
root = xr.concat(root_ds, dim=dim)
dtree = DataTree(data=root, name="root")
for grp in objs[0].groups[1:]:
ngrps = [obj[grp[1:]].ds for obj in objs]
ngrp = xr.concat(ngrps, dim=dim)
DataTree(ngrp, name=grp[1:], parent=dtree)
return dtree
[11]:
dsl = []
reindex_angle = dict(
tolerance=1.0, start_angle=0, stop_angle=360, angle_res=1.0, direction=1
)
for r, v in zip(volume_reflectivity, volume_velocity):
ds0 = [
xr.open_dataset(r0, engine="odim", group="sweep_0", reindex_angle=reindex_angle)
for r0 in r
]
ds1 = [
xr.open_dataset(v0, engine="odim", group="sweep_0", reindex_angle=reindex_angle)
for v0 in v
]
ds = [xr.merge([r0, v0], compat="override") for r0, v0 in zip(ds0, ds1)]
ds.insert(0, xr.open_dataset(r[0], group="/"))
dsl.append(ds)
# this takes some private functions from xradar, take care here
trees = [
DataTree(data=xradar.io.backends.common._assign_root(ds), name="root") for ds in dsl
]
trees = [
xradar.io.backends.common._attach_sweep_groups(tree, ds[1:])
for tree, ds in zip(trees, dsl)
]
vol = concat_radar_datatree(trees, dim="volume_time")
# align sweep_numbers to cover for single sweep single moment layout of DWD
for i, swp in enumerate(vol.groups[1:]):
vol[swp]["sweep_number"] = i
[12]:
vol
[12]:
<xarray.DatasetView> Dimensions: (volume_time: 6) Dimensions without coordinates: volume_time Data variables: volume_number (volume_time) int64 0 0 0 0 0 0 platform_type (volume_time) <U5 'fixed' 'fixed' ... 'fixed' 'fixed' instrument_type (volume_time) <U5 'radar' 'radar' ... 'radar' 'radar' time_coverage_start (volume_time) <U20 '2023-12-11T07:55:35Z' ... '2023-... time_coverage_end (volume_time) <U20 '2023-12-11T07:59:03Z' ... '2023-... longitude (volume_time) float64 6.967 6.967 6.967 ... 6.967 6.967 altitude (volume_time) float64 185.1 185.1 185.1 ... 185.1 185.1 latitude (volume_time) float64 51.41 51.41 51.41 ... 51.41 51.41 Attributes: Conventions: ODIM_H5/V2_2 version: None title: None institution: None references: None source: None history: None comment: im/exported using xradar instrument_name: None
[13]:
vol["sweep_9"]
[13]:
<xarray.DatasetView> Dimensions: (range: 240, azimuth: 360, volume_time: 6) Coordinates: * range (range) float32 125.0 375.0 625.0 ... 5.962e+04 5.988e+04 * azimuth (azimuth) float64 0.5 1.5 2.5 3.5 ... 357.5 358.5 359.5 elevation (volume_time, azimuth) float64 24.98 24.98 ... 24.98 time (volume_time, azimuth) datetime64[ns] 2023-12-11T07:58... longitude float64 6.967 latitude float64 51.41 altitude float64 185.1 Dimensions without coordinates: volume_time Data variables: DBZH (volume_time, azimuth, range) float32 -64.0 ... -64.0 sweep_mode (volume_time) <U20 'azimuth_surveillance' ... 'azimuth... sweep_number int64 9 prt_mode (volume_time) <U7 'not_set' 'not_set' ... 'not_set' follow_mode (volume_time) <U7 'not_set' 'not_set' ... 'not_set' sweep_fixed_angle (volume_time) float64 25.0 25.0 25.0 25.0 25.0 25.0 VRADH (volume_time, azimuth, range) float32 -128.0 ... -128.0
Inspect structure#
Root Group#
[14]:
vol.root
[14]:
<xarray.DatasetView> Dimensions: (volume_time: 6) Dimensions without coordinates: volume_time Data variables: volume_number (volume_time) int64 0 0 0 0 0 0 platform_type (volume_time) <U5 'fixed' 'fixed' ... 'fixed' 'fixed' instrument_type (volume_time) <U5 'radar' 'radar' ... 'radar' 'radar' time_coverage_start (volume_time) <U20 '2023-12-11T07:55:35Z' ... '2023-... time_coverage_end (volume_time) <U20 '2023-12-11T07:59:03Z' ... '2023-... longitude (volume_time) float64 6.967 6.967 6.967 ... 6.967 6.967 altitude (volume_time) float64 185.1 185.1 185.1 ... 185.1 185.1 latitude (volume_time) float64 51.41 51.41 51.41 ... 51.41 51.41 Attributes: Conventions: ODIM_H5/V2_2 version: None title: None institution: None references: None source: None history: None comment: im/exported using xradar instrument_name: None
Sweep Groups#
[15]:
vol["sweep_0"]
[15]:
<xarray.DatasetView> Dimensions: (range: 720, azimuth: 360, volume_time: 6) Coordinates: * range (range) float32 125.0 375.0 625.0 ... 1.796e+05 1.799e+05 * azimuth (azimuth) float64 0.5 1.5 2.5 3.5 ... 357.5 358.5 359.5 elevation (volume_time, azimuth) float64 5.493 5.493 ... 5.493 time (volume_time, azimuth) datetime64[ns] 2023-12-11T07:55... longitude float64 6.967 latitude float64 51.41 altitude float64 185.1 Dimensions without coordinates: volume_time Data variables: DBZH (volume_time, azimuth, range) float32 -64.0 ... -64.0 sweep_mode (volume_time) <U20 'azimuth_surveillance' ... 'azimuth... sweep_number int64 0 prt_mode (volume_time) <U7 'not_set' 'not_set' ... 'not_set' follow_mode (volume_time) <U7 'not_set' 'not_set' ... 'not_set' sweep_fixed_angle (volume_time) float64 5.499 5.499 5.499 5.499 5.499 5.499 VRADH (volume_time, azimuth, range) float32 -128.0 ... -128.0
plot sweeps#
DBZH#
[16]:
vol["sweep_0"].isel(volume_time=0)
[16]:
<xarray.DatasetView> Dimensions: (range: 720, azimuth: 360) Coordinates: * range (range) float32 125.0 375.0 625.0 ... 1.796e+05 1.799e+05 * azimuth (azimuth) float64 0.5 1.5 2.5 3.5 ... 357.5 358.5 359.5 elevation (azimuth) float64 5.493 5.493 5.493 ... 5.493 5.493 5.493 time (azimuth) datetime64[ns] 2023-12-11T07:55:54.763500288... longitude float64 6.967 latitude float64 51.41 altitude float64 185.1 Data variables: DBZH (azimuth, range) float32 -64.0 -64.0 ... -64.0 -64.0 sweep_mode <U20 'azimuth_surveillance' sweep_number int64 0 prt_mode <U7 'not_set' follow_mode <U7 'not_set' sweep_fixed_angle float64 5.499 VRADH (azimuth, range) float32 -128.0 6.008 ... -128.0 -128.0
[17]:
fig, gs = plt.subplots(
4, 3, figsize=(20, 30), sharex=True, sharey=True, constrained_layout=True
)
for i, grp in enumerate(vol.groups[1:]):
swp = vol[grp].isel(volume_time=0).ds
swp = swp.assign_coords(sweep_mode=swp.sweep_mode)
swp.DBZH.wrl.georef.georeference().wrl.vis.plot(ax=gs.flat[i], fig=fig)
ax = plt.gca()
ax.set_title(swp.sweep_fixed_angle.values)
fig.delaxes(gs.flat[-2])
fig.delaxes(gs.flat[-1])
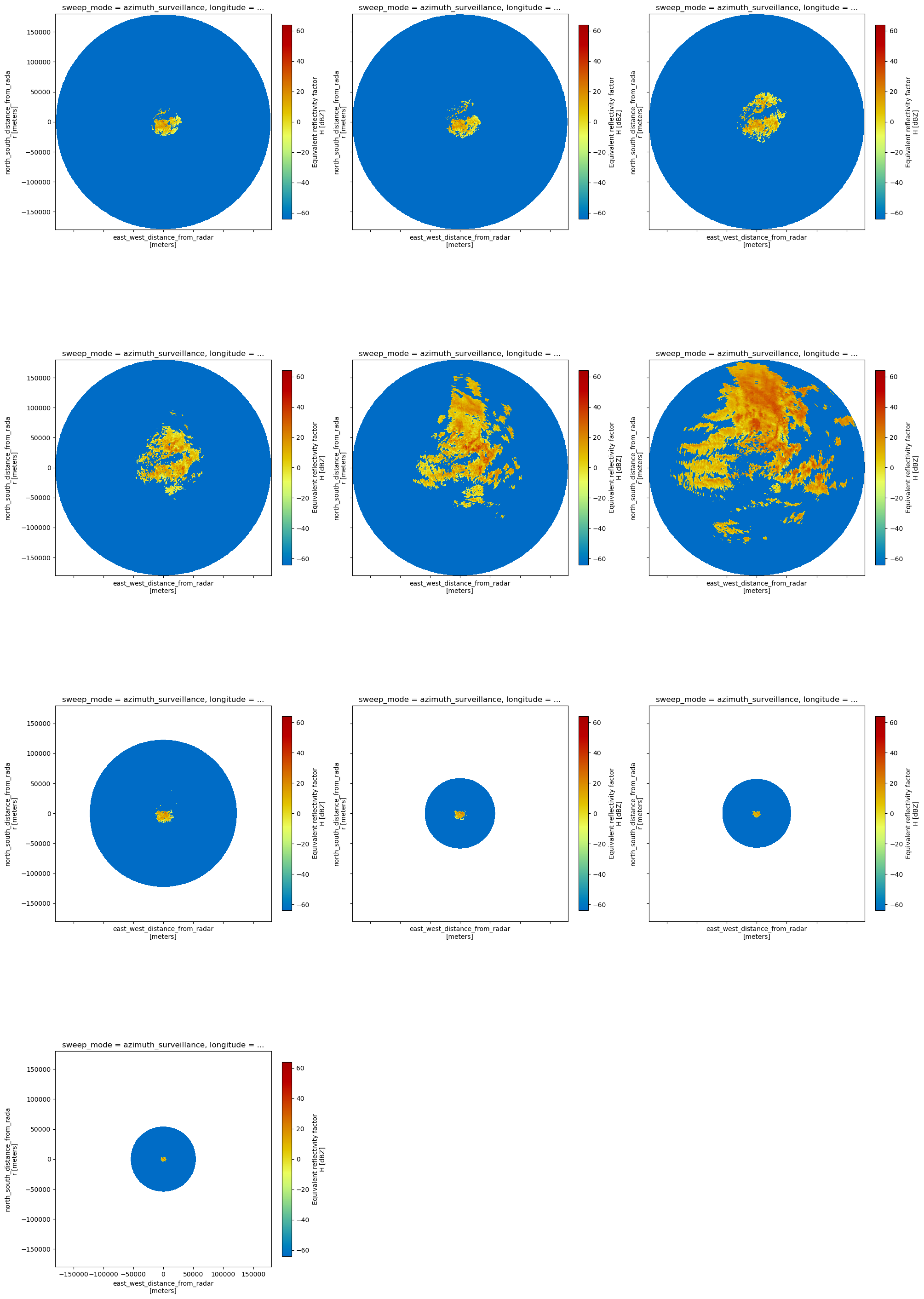
VRADH#
[18]:
fig, gs = plt.subplots(
4, 3, figsize=(20, 30), sharex=True, sharey=True, constrained_layout=True
)
for i, grp in enumerate(vol.groups[1:]):
swp = vol[grp].isel(volume_time=0).ds
swp = swp.assign_coords(sweep_mode=swp.sweep_mode)
swp.VRADH.wrl.georef.georeference().wrl.vis.plot(ax=gs.flat[i], fig=fig)
ax = plt.gca()
ax.set_title(swp.sweep_fixed_angle.values)
fig.delaxes(gs.flat[-2])
fig.delaxes(gs.flat[-1])
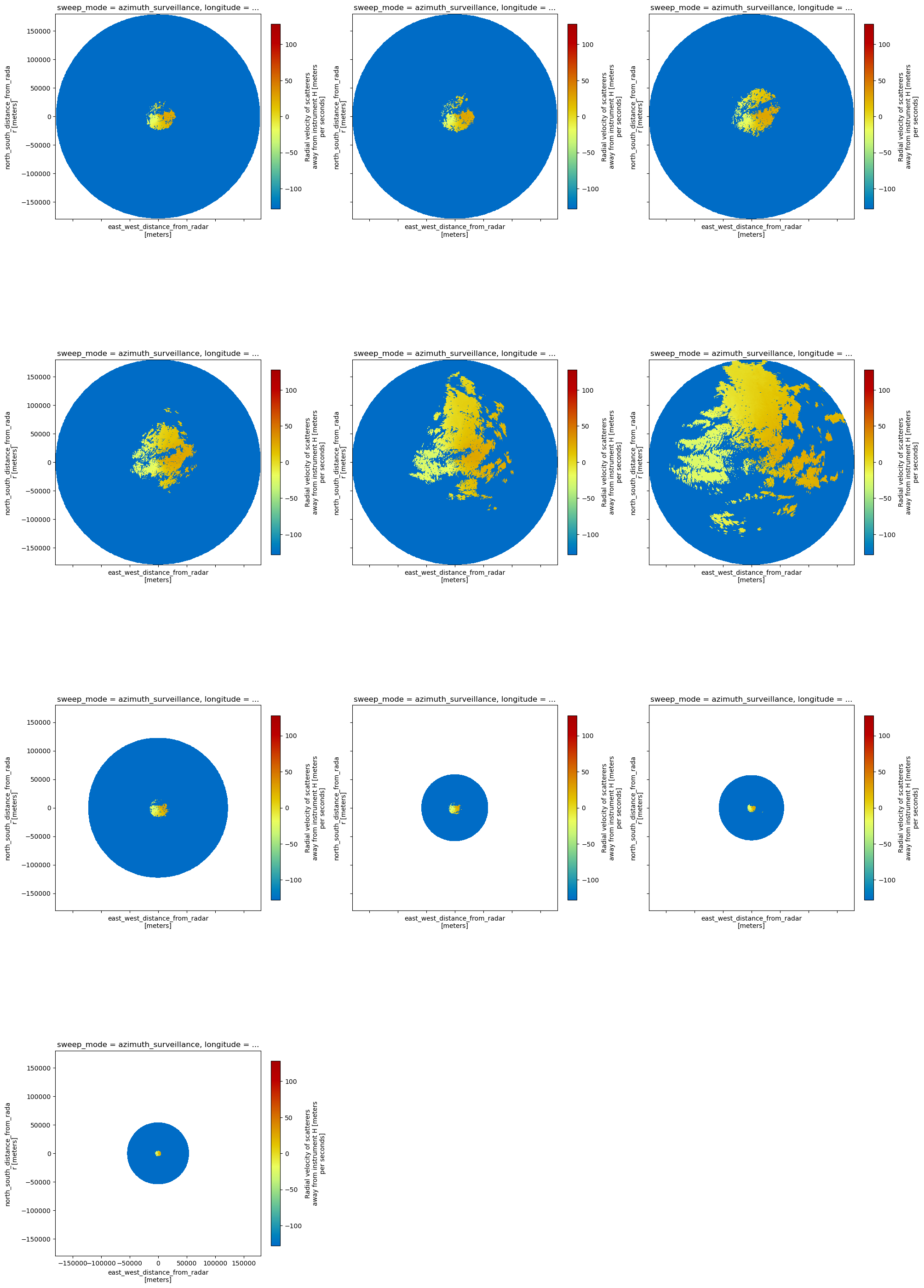
Plot single sweep using cartopy#
[19]:
vol0 = vol.isel(volume_time=0)
swp = vol0["sweep_9"].ds
# need to assign sweep_mode as coordinate
swp = swp.assign_coords(sweep_mode=swp.sweep_mode)
[20]:
import cartopy
import cartopy.crs as ccrs
import cartopy.feature as cfeature
map_trans = ccrs.AzimuthalEquidistant(
central_latitude=vol0.root.ds.latitude.values,
central_longitude=vol0.root.ds.longitude.values,
)
[21]:
map_proj = ccrs.AzimuthalEquidistant(
central_latitude=vol0.root.ds.latitude.values,
central_longitude=vol0.root.ds.longitude.values,
)
pm = swp.DBZH.wrl.georef.georeference().wrl.vis.plot(crs=map_proj)
ax = plt.gca()
ax.gridlines(crs=map_proj)
print(ax)
< GeoAxes: +proj=aeqd +ellps=WGS84 +lon_0=6.967111 +lat_0=51.405649 +x_0=0.0 +y_0=0.0 +no_defs +type=crs >
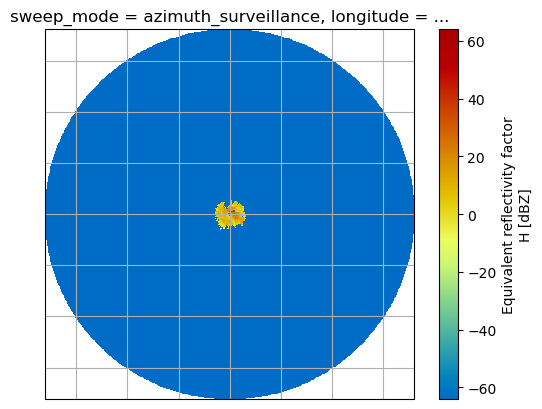
[22]:
map_proj = ccrs.Mercator(central_longitude=vol0.root.ds.longitude.values)
fig = plt.figure(figsize=(10, 8))
ax = fig.add_subplot(111, projection=map_proj)
pm = swp.DBZH.wrl.georef.georeference().wrl.vis.plot(ax=ax)
ax.gridlines(draw_labels=True)
[22]:
<cartopy.mpl.gridliner.Gridliner at 0x7ff768d3e390>
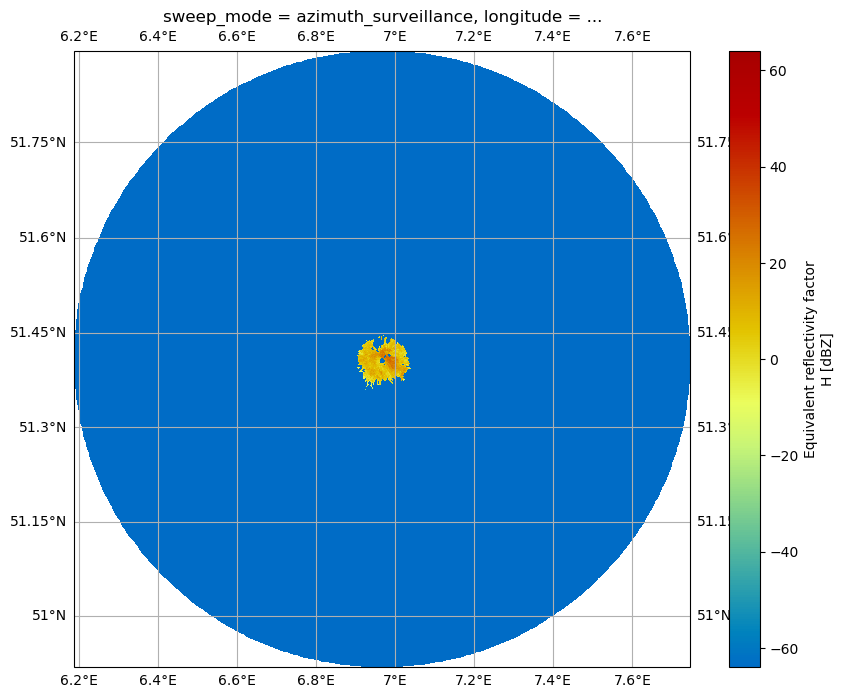
[23]:
fig = plt.figure(figsize=(10, 8))
proj = ccrs.AzimuthalEquidistant(
central_latitude=vol0.root.ds.latitude.values,
central_longitude=vol0.root.ds.longitude.values,
)
ax = fig.add_subplot(111, projection=proj)
pm = swp.DBZH.wrl.georef.georeference().wrl.vis.plot(ax=ax)
ax.gridlines()
[23]:
<cartopy.mpl.gridliner.Gridliner at 0x7ff768f9e610>
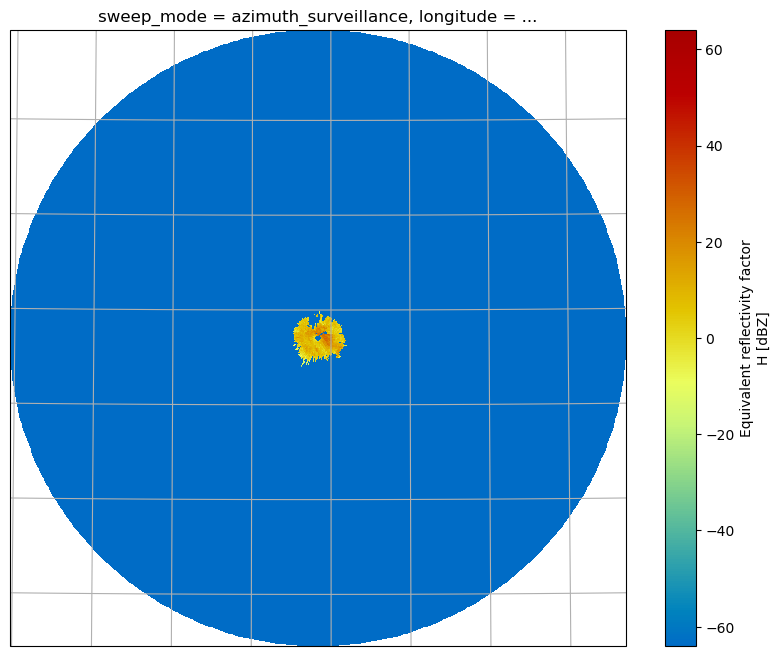
Inspect radar moments#
The DataArrays can be accessed by key or by attribute. Each DataArray inherits dimensions and coordinates of it’s parent dataset. There are attributes connected which are defined by Cf/Radial and/or ODIM_H5 standard.
[24]:
vol["sweep_9"].isel(volume_time=0).ds.DBZH
[24]:
<xarray.DataArray 'DBZH' (azimuth: 360, range: 240)> array([[-64.00293 , -64.00293 , 23.176842, ..., -64.00293 , -64.00293 , -64.00293 ], [-64.00293 , -64.00293 , 22.822334, ..., -64.00293 , -64.00293 , -64.00293 ], [-64.00293 , -64.00293 , 22.230507, ..., -64.00293 , -64.00293 , -64.00293 ], ..., [-64.00293 , -64.00293 , 23.335052, ..., -64.00293 , -64.00293 , -64.00293 ], [-64.00293 , -64.00293 , 19.271393, ..., -64.00293 , -64.00293 , -64.00293 ], [-64.00293 , -64.00293 , 22.318405, ..., -64.00293 , -64.00293 , -64.00293 ]], dtype=float32) Coordinates: * range (range) float32 125.0 375.0 625.0 ... 5.962e+04 5.988e+04 * azimuth (azimuth) float64 0.5 1.5 2.5 3.5 4.5 ... 356.5 357.5 358.5 359.5 elevation (azimuth) float64 24.98 24.98 24.98 24.98 ... 24.98 24.98 24.98 time (azimuth) datetime64[ns] 2023-12-11T07:58:54.953000192 ... 202... longitude float64 6.967 latitude float64 51.41 altitude float64 185.1 Attributes: _Undetect: 0.0 units: dBZ standard_name: radar_equivalent_reflectivity_factor_h long_name: Equivalent reflectivity factor H
[25]:
vol["sweep_9"].isel(volume_time=0).ds.sweep_mode
[25]:
<xarray.DataArray 'sweep_mode' ()> array('azimuth_surveillance', dtype='<U20') Coordinates: longitude float64 6.967 latitude float64 51.41 altitude float64 185.1
Plot Quasi Vertical Profile#
[26]:
ts = vol["sweep_9"]
ts
[26]:
<xarray.DatasetView> Dimensions: (range: 240, azimuth: 360, volume_time: 6) Coordinates: * range (range) float32 125.0 375.0 625.0 ... 5.962e+04 5.988e+04 * azimuth (azimuth) float64 0.5 1.5 2.5 3.5 ... 357.5 358.5 359.5 elevation (volume_time, azimuth) float64 24.98 24.98 ... 24.98 time (volume_time, azimuth) datetime64[ns] 2023-12-11T07:58... longitude float64 6.967 latitude float64 51.41 altitude float64 185.1 Dimensions without coordinates: volume_time Data variables: DBZH (volume_time, azimuth, range) float32 -64.0 ... -64.0 sweep_mode (volume_time) <U20 'azimuth_surveillance' ... 'azimuth... sweep_number int64 9 prt_mode (volume_time) <U7 'not_set' 'not_set' ... 'not_set' follow_mode (volume_time) <U7 'not_set' 'not_set' ... 'not_set' sweep_fixed_angle (volume_time) float64 25.0 25.0 25.0 25.0 25.0 25.0 VRADH (volume_time, azimuth, range) float32 -128.0 ... -128.0
[27]:
fig = plt.figure(figsize=(10, 4))
ax = fig.add_subplot(111)
ts.ds.DBZH.median("azimuth").plot(x="volume_time", vmin=-10, vmax=30, ax=ax)
ax.set_title(f"{np.datetime_as_string(ts.ds.time[0][0].values, unit='D')}")
ax.set_ylim(0, 20000)
[27]:
(0.0, 20000.0)
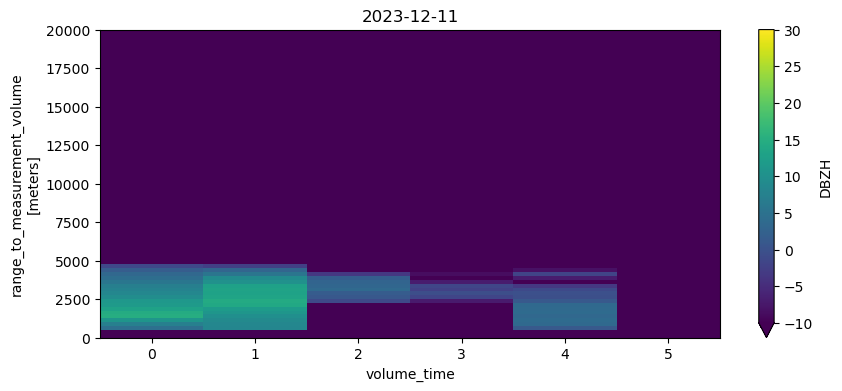
Export to OdimH5#
This exports the radar volume at given timestep including all moments into one ODIM_H5 compliant data file.
[28]:
xradar.io.to_odim(vol0, "dwd_odim.h5", source="RAD:DWD")
Export to Cf/Radial2#
This exports the radar volume at given timestep including all moments into one Cf/Radial2 compliant data file.
[29]:
xradar.io.to_cfradial2(vol0, "dwd_cfradial2.nc")
Import again#
[30]:
vol1 = xradar.io.open_odim_datatree("dwd_odim.h5")
display(vol1)
<xarray.DatasetView> Dimensions: () Data variables: volume_number int64 0 platform_type <U5 'fixed' instrument_type <U5 'radar' time_coverage_start <U20 '2023-12-11T07:55:36Z' time_coverage_end <U20 '2023-12-11T07:59:02Z' longitude float64 6.967 altitude float64 185.1 latitude float64 51.41 Attributes: Conventions: ODIM_H5/V2_2 version: None title: None institution: None references: None source: None history: None comment: im/exported using xradar instrument_name: None
[31]:
vol2 = open_datatree("dwd_cfradial2.nc")
display(vol2)
<xarray.DatasetView> Dimensions: () Data variables: volume_number int64 ... platform_type <U5 ... instrument_type <U5 ... time_coverage_start <U20 ... time_coverage_end <U20 ... longitude float64 ... altitude float64 ... latitude float64 ... Attributes: Conventions: Cf/Radial version: 2.0 title: None institution: None references: None source: None history: None: xradar v0.4.2 CfRadial2 export comment: im/exported using xradar instrument_name: None