Quick-view a RHI sweep in polar or cartesian reference systems#
[1]:
import numpy as np
import matplotlib.pyplot as plt
import wradlib as wrl
import warnings
warnings.filterwarnings("ignore")
try:
get_ipython().run_line_magic("matplotlib inline")
except:
plt.ion()
/home/runner/micromamba/envs/wradlib-tests/lib/python3.11/site-packages/h5py/__init__.py:36: UserWarning: h5py is running against HDF5 1.14.3 when it was built against 1.14.2, this may cause problems
_warn(("h5py is running against HDF5 {0} when it was built against {1}, "
Read a RHI polar data set from University Bonn XBand radar#
[2]:
filename = wrl.util.get_wradlib_data_file("hdf5/2014-06-09--185000.rhi.mvol")
data1, metadata = wrl.io.read_gamic_hdf5(filename)
img = data1["SCAN0"]["ZH"]["data"]
# mask data array for better presentation
mask_ind = np.where(img <= np.nanmin(img))
img[mask_ind] = np.nan
img = np.ma.array(img, mask=np.isnan(img))
r = metadata["SCAN0"]["r"]
th = metadata["SCAN0"]["el"]
print(th.shape)
az = metadata["SCAN0"]["az"]
site = (
metadata["VOL"]["Longitude"],
metadata["VOL"]["Latitude"],
metadata["VOL"]["Height"],
)
img = wrl.georef.create_xarray_dataarray(
img, r=r, phi=az, theta=th, site=site, dim0="elevation", sweep_mode="rhi"
)
img
(450,)
[2]:
<xarray.DataArray (elevation: 450, range: 667)> array([[ 4.3487625, 15.684074 , 14.629356 , ..., 128.00293 , 128.00293 , 128.00293 ], [ 3.7921066, 15.830559 , 13.624435 , ..., 128.00293 , 128.00293 , 128.00293 ], [ 3.8712082, 15.754387 , 12.074585 , ..., 128.00293 , 128.00293 , 128.00293 ], ..., [ 4.471817 , 16.114746 , 14.95163 , ..., 128.00293 , 128.00293 , 128.00293 ], [ 4.694481 , 15.72802 , 14.397903 , ..., 128.00293 , 128.00293 , 128.00293 ], [ 4.9376526, 16.059082 , 14.227974 , ..., 128.00293 , 128.00293 , 128.00293 ]], dtype=float32) Coordinates: * range (range) float64 75.0 150.0 225.0 ... 4.995e+04 5.002e+04 azimuth (elevation) float64 225.0 225.0 225.0 ... 225.0 225.0 225.0 * elevation (elevation) float64 0.2 0.3 0.5 0.7 0.9 ... 89.3 89.5 89.7 89.9 longitude float64 7.072 latitude float64 50.73 altitude float64 99.5 sweep_mode <U3 'rhi'
Inspect the data set a little
[3]:
print("Shape of polar array: %r\n" % (img.shape,))
print("Some meta data of the RHI file:")
print("\tdatetime: %r" % (metadata["SCAN0"]["Time"],))
Shape of polar array: (450, 667)
Some meta data of the RHI file:
datetime: '2014-06-09T18:50:01.000Z'
The simplest way to plot this dataset#
[4]:
img = img.wrl.georef.georeference()
pm = img.wrl.vis.plot()
txt = plt.title("Simple RHI - Rays/Bins")
# plt.gca().set_xlim(0,100000)
# plt.gca().set_ylim(0,100000)
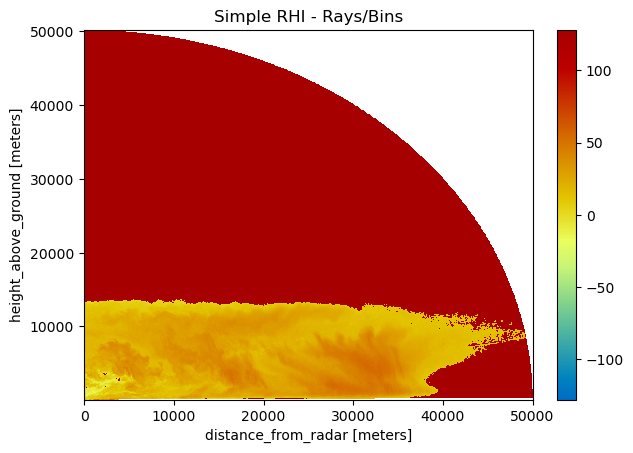
[5]:
pm = img.wrl.vis.plot()
plt.gca().set_ylim(0, 15000)
txt = plt.title("Simple RHI - Rays/Bins - with ylimits")
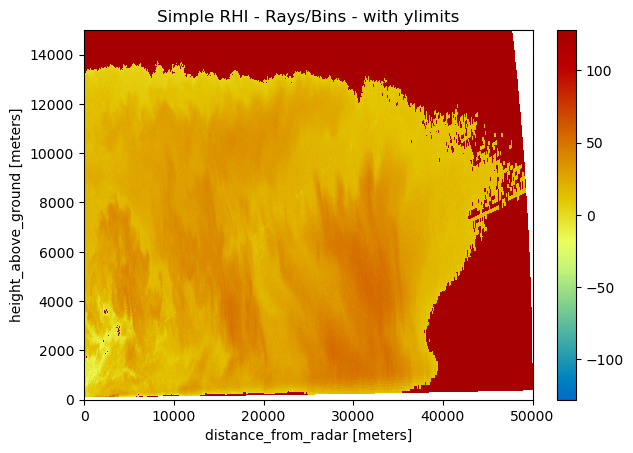
[6]:
pm = img.wrl.vis.plot(crs="cg")
plt.gca().set_title("Curvelineart Grid RHI", y=1.0, pad=20)
[6]:
Text(0.5, 1.0, 'Curvelineart Grid RHI')
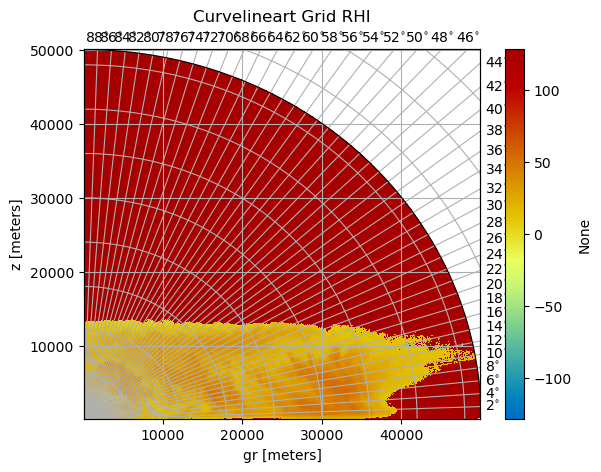
More decorations and annotations#
You can annotate these plots by using standard matplotlib methods.
[7]:
pm = img.wrl.vis.plot()
ax = plt.gca()
ylabel = ax.set_xlabel("Ground Range [m]")
ylabel = ax.set_ylabel("Height [m]")
title = ax.set_title("RHI manipulations/colorbar", y=1, pad=20)
# you can now also zoom - either programmatically or interactively
xlim = ax.set_xlim(25000, 40000)
ylim = ax.set_ylim(0, 15000)
# as the function returns the axes- and 'mappable'-objects colorbar needs, adding a colorbar is easy
cb = plt.colorbar(pm, ax=ax)
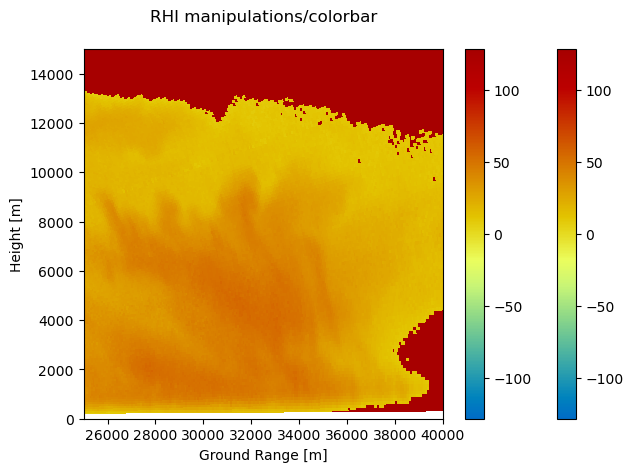